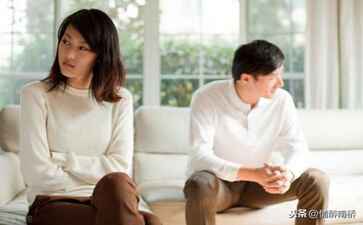
振安區遍闊插座有限公司位于南昌縣,振安區遍闊插座有限公司www.notekit.cn經營范圍含:字畫、廣電、服飾、廣告材料、物業保潔、建筑設計、種牛、電氣、服裝加工設備、毛皮服裝(依法須經批準的項目,經相關部門批準后方可開展經營活動)。。
振安區遍闊插座有限公司將堅定不移地貫徹落實黨中央和國務院關于國有企業深化改革的重要決策部署,緊密遵循國資委關于壯大和強化國有企業的具體要求。在此基礎上,我們將持續推進企業的深化改革進程,致力于進一步優化產業結構,實現資源的科學配置,從而有效提升企業的核心競爭力。我們還將致力于全面提升企業的整體素質,使其能夠更好地適應并應對國際與國內市場的挑戰與機遇。我們將瞄準更為遠大的目標,不懈奮斗,努力將企業發展推向新的高度。
振安區遍闊插座有限公司在發展中注重與業界人士合作交流,強強聯手,共同發展壯大。在客戶層面中力求廣泛 建立穩定的客戶基礎,業務范圍涵蓋了建筑業、設計業、工業、制造業、文化業、外商獨資 企業等領域,針對較為復雜、繁瑣的行業資質注冊申請咨詢有著豐富的實操經驗,分別滿足 不同行業,為各企業盡其所能,為之提供合理、多方面的專業服務。
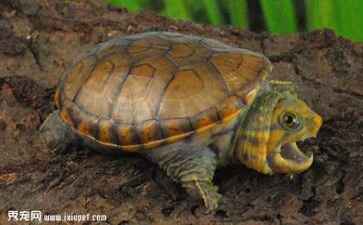
振安區遍闊插座有限公司秉承“質量為本,服務社會”的原則,立足于高新技術,科學管理,擁有現代化的生產、檢測及試驗設備,已建立起完善的產品結構體系,產品品種,結構體系完善,性能質量穩定。
振安區遍闊插座有限公司是一家具有完整生態鏈的企業,它為客戶提供綜合的、專業現代化裝修解決方案。為消費者提供較優質的產品、較貼切的服務、較具競爭力的營銷模式。
核心價值:尊重、誠信、推崇、感恩、合作
經營理念:客戶、誠信、專業、團隊、成功
服務理念:真誠、專業、精準、周全、可靠
企業愿景:成為較受信任的創新性企業服務開放平臺
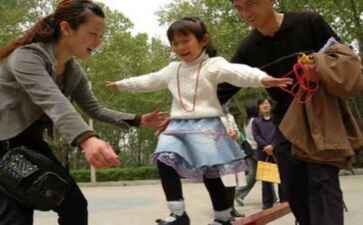